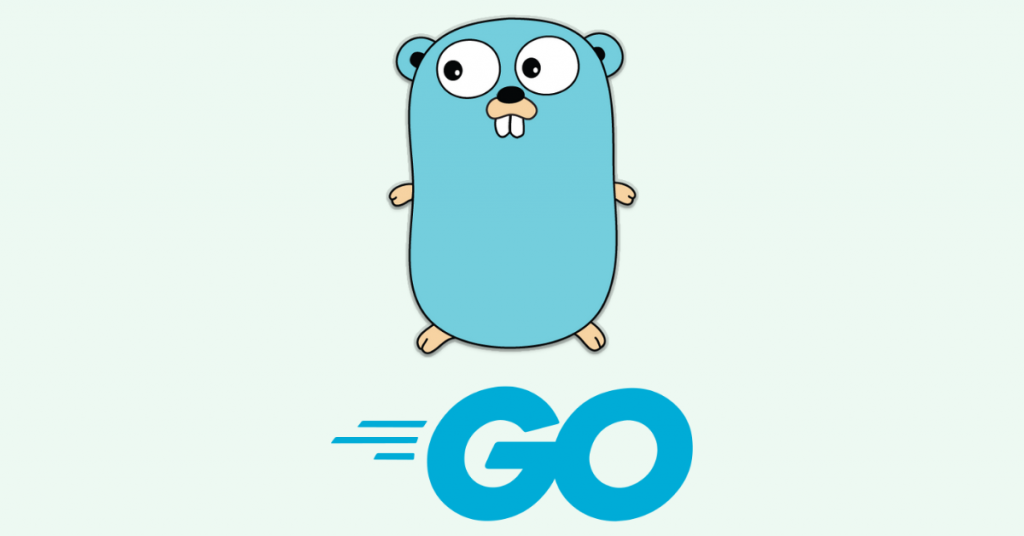
Go by Example :Goroutines.
A gorountine is a lightweight thread of execution .
package main
import (
"fmt"
"sync"
"time"
)
func f(from string) {
for i :=0; i <4; i++ {
fmt.Println(from, ",from:", i)
}
}
func main() {
f("direct")
gof("goroutines")
gofunc(msgstring) {
fmt.Println(time.Now().Format("2006-01-02 15:04:05"), msg)
}("going--going--going")
time.Sleep(time.Second)
fmt.Println("done...")
//waitGroup.
var wg sync.WaitGroup
wg.Add(1)
gofunc() {
defer wg.Done()
fmt.Println("I'm a GoRoutines.....")
}()
wg.Wait()
fmt.Println("main func end....")
return
}
Suppose we have a function call f(s). Here’s how we’d call that in the usual way ,running it synchronously.
To invoke this function in a goroutine, use go f(s). This new goroutine will execute concurrently with the calling one.
You can also start a goroutine for an anonymous function call .
Our two function calls are running asynchronously in separate goroutines now . Wait for them to finish (for a more robust approach , use a WaitGroup).
When we run this program , we see the output of the blocking call first , then the output of the two goroutines. The goroutings’ output may be interleaved , because goroutines are being run concurrently by the Go runtime.